Bip 38 bitcoin exchange
24 comments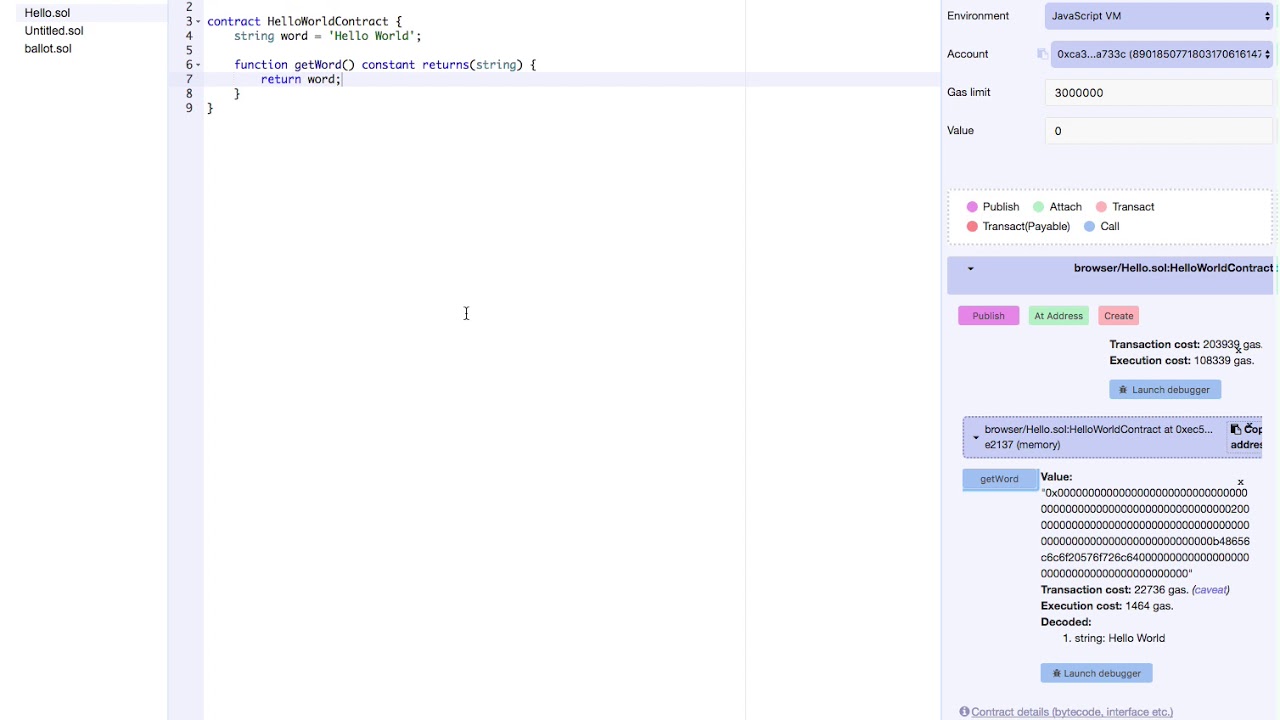
Bitcoin money adder download free
This page will help you build a Hello, World contract on the ethereum command line. If you don't know how to use the command line we recommend you skip this tutorial and instead build a Custom token using the graphical user interface. Smart contracts are account holding objects on the ethereum blockchain. They contain code functions and can interact with other contracts, make decisions, store data, and send ether to others. Contracts are defined by their creators, but their execution, and by extension the services they offer, is provided by the ethereum network itself.
They will exist and be executable as long as the whole network exists, and will only disappear if they were programmed to self destruct. What can you do with contracts? Well, you can do almost anything really, but for our getting started guide let's do some simple things: To start you will create a classic "Hello World" contract, then you can build your own crypto token to send to whomever you like. Once you've mastered that then you will raise funds through a crowdfunding that, if successful, will supply a radically transparent and democratic organization that will only obey its own citizens, will never swerve away from its constitution and cannot be censored or shut down.
And all that in less than lines of code. Please confirm that the GUI is closed before entering the geth console. Run geth to begin the sync process this may take a while on the first run. The Frontier is a big open territory and sometimes you might feel lonely, so our first order of business will be to create a little automatic companion to greet you whenever you feel lonely.
The Greeter is an intelligent digital entity that lives on the blockchain and is able to have conversations with anyone who interacts with it, based on its input. Here is its code:. You'll notice that there are two different contracts in this code: This is because Solidity the high level contract language we are using has inheritance , meaning that one contract can inherit characteristics of another.
This is very useful to simplify coding as common traits of contracts don't need to be rewritten every time, and all contracts can be written in smaller, more readable chunks. So by just declaring that greeter is mortal you inherited all characteristics from the "mortal" contract and kept the greeter code simple and easy to read. The inherited characteristic "mortal" simply means that the greeter contract can be killed by its owner, to clean up the blockchain and recover funds locked into it when the contract is no longer needed.
Contracts in ethereum are, by default, immortal and have no owner, meaning that once deployed the author has no special privileges anymore. Consider this before deploying. You can get both of these by using a Solidity compiler.
If you have not installed a compiler, you can either:. If you installed the compiler on your machine, you need to compile the contract to acquire the compiled code and Application Binary Interface. This will create two files, one file containing the compiled code and one file creating the Application Binary Interface in a directory called target. You will see that there are files created for both contracts; but because Greeter includes Mortal you do not need to deploy Mortal to deploy Greeter.
You have now compiled your code and made it available to Geth. Now you need to get it ready for deployment, this includes setting some variables up, like what greeting you want to use. Edit the first line below to something more interesting than "Hello World!
If you don't have Solc installed, you can simply use the online IDE. Copy the source code at the top of this page to Remix and it should automatically compile your code. You can safely ignore any yellow warning boxes on the right plane. To access the compiled code, ensure that the dropdown menu on the right pane has greeter selected. Then click on the Details button directly to the right of the dropdown.
Create a temporary text file on your computer and paste that code. Make sure to change the first line to look like the following:. Now you can paste the resulting text on your geth window, or import the file with loadScript "yourFilename. Wait up to thirty seconds and you'll see a message like this:. You may have to "unlock" the account that is sending the transaction using the password you picked in the beginning, because you need to pay for the gas costs to deploying your contract: There are many useful stats, including the latest gas prices at the network stats page.
Notice that the cost is not paid to the ethereum developers , instead it goes to the Miners , those peers whose computers are working to find new blocks and keep the network secure.
Gas price is set by the market of the current supply and demand of computation. If the gas prices are too high, you can become a miner and lower your asking price. Within less than a minute, you should have a log with the contract address, this means you've successfully deployed your contract. You can verify the deployed code which will be compiled by using this command:. If it returns anything other than "0x" then congratulations! Your little Greeter is live! If the contract is created again by performing another eth.
Since this call changes nothing on the blockchain, it returns instantly and without any gas cost. You should see it return your greeting:. If you compiled the code using Remix , the last line of code above won't work for you! On the right pane, click on the Details button and scroll down to the ABI textbox. Click on the copy button to copy the entire ABI, then paste it in a temporary text document. Then you can instantiate a JavaScript object which can be used to call the contract on any machine connected to the network.
Of course, greeterAddress must be replaced with your contract's unique address. You must be very excited to have your first contract live, but this excitement wears off sometimes, when the owners go on to write further contracts, leading to the unpleasant sight of abandoned contracts on the blockchain.
In the future, blockchain rent might be implemented in order to increase the scalability of the blockchain but for now, be a good citizen and humanely put down your abandoned bots.
A transaction will need to be sent to the network and a fee to be paid for the changes made to the blockchain after the code below is run.
The self-destruct is subsidized by the network so it will cost much less than a usual transaction. This can only be triggered by a transaction sent from the contracts owner.
You can verify that the deed is done simply seeing if this returns Notice that every contract has to implement its own kill clause. In this particular case only the account that created the contract can kill it. If you don't add any kill clause it could potentially live forever independently of you and any earthly borders, so before you put it live check what your local laws say about it, including any possible limitation on technology export, restrictions on speech and maybe any legislation on the civil rights of sentient digital beings.
Treat your bots humanely. Create a tradeable digital token that can be used as a currency, a representation of an asset, a virtual share, a proof of membership or anything at all. These tokens use a standard coin API so your contract will be automatically compatible with any wallet, other contract or exchange also using this standard.
The total amount of tokens in circulation can be set to a simple fixed amount or fluctuate based on any programmed ruleset. Building a smart contract using the command line This page will help you build a Hello, World contract on the ethereum command line. So let's start now. Here is its code: Compiling your contract using the Solc Compiler Before you are able to deploy your contract, you'll need two things: The compiled code The Application Binary Interface, which is a JavaScript Object that defines how to interact with the contract You can get both of these by using a Solidity compiler.
If you have not installed a compiler, you can either: You can use these two files to create and deploy the contract. Make sure to change the first line to look like the following: Wait up to thirty seconds and you'll see a message like this: You can verify the deployed code which will be compiled by using this command: Run the Greeter In order to call your bot, just type the following command in your terminal: You should see it return your greeting: The Address where the contract is located The ABI Application Binary Interface , which is a sort of user manual describing the name of the contract's functions and how to call them to your JavaScript console To get the Address , run this command: Cleaning up after yourself: You can verify that the deed is done simply seeing if this returns 0: Design and issue your own cryptocurrency Create a tradeable digital token that can be used as a currency, a representation of an asset, a virtual share, a proof of membership or anything at all.
A tradeable token with a fixed supply A central bank that can issue money A puzzle-based cryptocurrency.